Erzeugung eines Dokuments
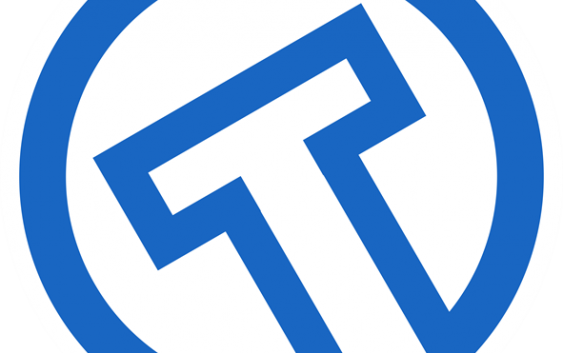
Hier stellen wir Ihnen ein ABAP-Programm vor, mit dem ein Word-Dokument erzeugt und mit Daten “gefüttert” wird.
Mit diesem Programm stellen wir Ihnen eine Möglichkeit vor, wie Sie aus SAP heraus ein Word-Dokument erzeugen und mit Daten füllen können.
Es werden sowohl Tabellen erzeugt und befüllt als auch Formatierungen vorgenommen.
Das Beispiel erzeugt ein Dokument mit Kopfzeile und Tabellen. Es sieht nicht sehr schön aus, aber es zeigt die Anwendung der wichtigsten Methoden.
So sieht’s aus:
|
Das Coding
REPORT zz_word .
**********************************************************************
*** ***
*** https://tricktresor.com ***
*** ***
**********************************************************************
*——————————————————————–*
*
* Starten von Word und Übergabe von Daten, Erzeugung von Tabellen,
* Positionieren innerhalb der Tabellen und Formatierung von Text bzw.
* Absätzen
*
* ACHTUNG:
* Ab der Version 4.7 muss die Schriftgröße als Integer
* übergeben werden!
*——————————————————————–*
*** Benötigte Typinformationen für OLE
INCLUDE ole2incl.
INCLUDE docsincl.
*** Programmvariablen
DATA:
BEGIN OF t_item OCCURS 0,
posnr TYPE posnr,
matnr TYPE matnr,
mtext(40) TYPE c,
menge(6) TYPE p,
meins TYPE meins,
netpr(6) TYPE p DECIMALS 2,
waers TYPE waers,
END OF t_item,
l_menge(20),
l_preis(20),
l_text(80),
h_word TYPE ole2_object.
START-OF-SELECTION.
PERFORM word_starten.
*———————————————————————*
* FORM WORD_STARTEN
*———————————————————————*
FORM word_starten.
IF h_word-header = space OR h_word-handle EQ -1.
*** Word starten
CREATE OBJECT h_word ‘Word.Basic’.
CALL METHOD OF h_word ‘FileNew’
EXPORTING #1 = ‘normal.dot’.
CALL METHOD OF h_word ‘AppShow’.
*** Kopfzeile erzeugen
PERFORM kopfzeile.
*** Infotext
PERFORM infotext.
*** Testdaten für die Positionstabelle generieren
PERFORM testdaten.
IF NOT t_item[] IS INITIAL.
*** Überschirften und Tabelle
PERFORM item_title.
ENDIF.
LOOP AT t_item.
*** Ausgabe der Positionen
PERFORM ausgabe_position.
ENDLOOP.
*** Ausgabe der restlichen Informationen
PERFORM ende.
ENDIF.
IF sy-subrc NE 0.
WRITE: / ‘Error when opening word.application’, sy-msgli.
ENDIF.
FREE OBJECT h_word.
h_word-handle = -1.
ENDFORM.
*———————————————————————*
* FORM WRITE_TEXT *
*———————————————————————*
* –> TEXT
* –> FONT
* –> SIZE
* –> LOOK
*———————————————————————*
FORM write_text USING text font size look.
CALL METHOD OF h_word ‘Font’ EXPORTING #01 = font.
CALL METHOD OF h_word ‘FontSize’ EXPORTING #1 = size.
IF look NE ”.
CALL METHOD OF h_word look.
ENDIF.
CALL METHOD OF h_word ‘Insert’ EXPORTING #1 = text.
ENDFORM.
*———————————————————————*
* FORM item_title *
*———————————————————————*
* Aufbau und Füllen der Positionstabelle
*———————————————————————*
FORM item_title.
CALL METHOD OF h_word ‘TableInsertTable’
EXPORTING #1 = ‘ ‘
#2 = ‘2’ “Spalten
#3 = ‘8’ “Zeilen
#4 = ‘Auto’.
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘9,0 cm’. “Breite der 1. Spalte
PERFORM write_text
USING ‘Adresse:’ ‘TimesNewRoman’ ’14’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
PERFORM write_text
USING ‘Informationen:’ ‘TimesNewRoman’ ’14’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
PERFORM write_text
USING ‘Tricktresor’ ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
PERFORM write_text
USING ‘Information 1’ ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
PERFORM write_text
USING ‘Im Banksafe 2’ ‘TimesNewRoman’ ’10’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
PERFORM write_text
USING ‘Information 2’ ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
PERFORM write_text
USING ‘Information 3’ ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
PERFORM write_text
USING ‘12345 Klein-Schlüssel’ ‘TimesNewRoman’ ’10’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
PERFORM write_text
USING ‘Information 4’ ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘EndOfDocument’.
CALL METHOD OF h_word ‘EndOfLine’.
CALL METHOD OF h_word ‘InsertPara’.
CALL METHOD OF h_word ‘TableInsertTable’
EXPORTING #1 = ‘ ‘
#2 = ‘6’
#3 = ‘1’
#4 = ‘Auto’.
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘2,0 cm’.
PERFORM write_text
USING ‘Material number’ ‘TimesNewRoman’ ’12’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘4,0 cm’.
PERFORM write_text
USING ‘Material description’
‘TimesNewRoman’ ’12’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘2,1 cm’.
PERFORM write_text
USING ‘Quantity’ ‘TimesNewRoman’ ’12’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘1,6 cm’.
PERFORM write_text
USING ‘ Unit’ ‘TimesNewRoman’ ’12’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘3,0 cm’.
CALL METHOD OF h_word ‘RightPara’ EXPORTING #01 = ‘AlignRight’.
PERFORM write_text
USING ‘Price / Unit’ ‘TimesNewRoman’ ’12’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘3,2 cm’.
CALL METHOD OF h_word ‘RightPara’
EXPORTING #01 = ‘AlignRight’.
PERFORM write_text
USING ‘Net value’ ‘TimesNewRoman’ ’12’ ‘Bold’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
ENDFORM.
*&———————————————————————*
*& Form kopfzeile
*&———————————————————————*
FORM kopfzeile.
CALL METHOD OF h_word ‘ViewHeader’.
*** Anzeige Tabellengitter (0=aus)
CALL METHOD OF h_word ‘TableGridLines’
EXPORTING #1 = ‘1’.
CALL METHOD OF h_word ‘TableInsertTable’
EXPORTING #1 = ‘ ‘
#2 = ‘2’
#3 = ‘1’
#4 = ‘Auto’.
*** Kopftext links schreiben
PERFORM write_text
USING ‘TRICKTRESOR’ ‘TimesNewRoman’ ’24’ ‘Bold’.
*** Kopftext links mit Absatzformat “Überschrift 1” formatieren
CALL METHOD OF h_word ‘FormatStyle’
EXPORTING #1 = ‘Überschrift 1’.
*** Breite der Tabellenspalte ändern
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘9,0 cm’.
CALL METHOD OF h_word ‘NextCell’.
*** Logo einbinden
CALL METHOD OF h_word ‘InsertPicture’
EXPORTING #1 = ‘C:/temp/logo.bmp’.
PERFORM write_text
USING ‘DEMO’ ‘TimesNewRoman’ ’24’ ‘Bold’.
*** Kopffenster wieder schließen
CALL METHOD OF h_word ‘CloseViewHeaderFooter’.
ENDFORM. ” kopfzeile
*&———————————————————————*
*& Form infotext
*&———————————————————————*
FORM infotext.
CALL METHOD OF h_word ‘TableInsertTable’
EXPORTING #1 = ‘ ‘
#2 = ‘2’
#3 = ‘1’
#4 = ‘Auto’.
CALL METHOD OF h_word ‘TableColumnWidth’
EXPORTING #01 = ‘9,0 cm’.
PERFORM write_text
USING ‘Dieses Dokument wurde aus R/3 erzeugt’
‘TimesNewRoman ‘
’12’
”.
*** Absatz linksbündig
CALL METHOD OF h_word ‘LeftPara’.
CALL METHOD OF h_word ‘NextCell’.
*** Datum übergeben
write sy-datum to l_text.
PERFORM write_text
USING l_text ‘TimesNewRoman’ ’10’ ‘Italic’.
*** Datum rechtsbündig
CALL METHOD OF h_word ‘RightPara’.
CALL METHOD OF h_word ‘EndOfDocument’.
CALL METHOD OF h_word ‘EndOfLine’.
CALL METHOD OF h_word ‘InsertPara’.
ENDFORM. ” infotext
*&———————————————————————*
*& Form testdaten
*&———————————————————————*
FORM testdaten.
CLEAR t_item.
t_item-posnr = 1.
t_item-matnr = ‘ABCD’.
t_item-mtext = ‘Testartikel ABCD groß’.
t_item-menge = ’10’.
t_item-meins = ‘ST’.
t_item-netpr = ‘21.45’.
t_item-waers = ‘EUR’.
APPEND t_item.
t_item-posnr = 2.
t_item-matnr = ‘TEST123’.
t_item-mtext = ‘Testartikel 123 klein’.
t_item-menge = ‘2000’.
t_item-meins = ‘ST’.
t_item-netpr = ‘11.45’.
t_item-waers = ‘EUR’.
APPEND t_item.
ENDFORM. ” testdaten
*&———————————————————————*
*& Form ausgabe_position
*&———————————————————————*
FORM ausgabe_position.
PERFORM write_text USING t_item-matnr ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
PERFORM write_text USING t_item-mtext ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
WRITE t_item-menge TO l_menge UNIT t_item-meins.
PERFORM write_text USING l_menge ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
PERFORM write_text USING t_item-meins ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
CALL METHOD OF h_word ‘RightPara’
EXPORTING #01 = ‘AlignRight’.
WRITE t_item-netpr TO l_preis CURRENCY t_item-waers.
PERFORM write_text USING l_preis ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
CALL METHOD OF h_word ‘RightPara’
EXPORTING #01 = ‘AlignRight’.
l_text = t_item-menge * t_item-netpr.
WRITE l_text TO l_text CURRENCY t_item-waers.
PERFORM write_text USING l_text ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NextCell’.
CALL METHOD OF h_word ‘NormalStyle’.
ENDFORM. ” ausgabe_position
*&———————————————————————*
*& Form ende
*&———————————————————————*
FORM ende.
*** Ans Ende vom Dokument springen
CALL METHOD OF h_word ‘EndOfDocument’.
CALL METHOD OF h_word ‘InsertPara’.
PERFORM write_text
USING ‘Dieses Programm’ ‘TimesNewRoman’ ’14’ ‘Bold’.
CALL METHOD OF h_word ‘InsertPara’.
PERFORM write_text
USING ‘wurde Ihnen präsentiert’ ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘InsertPara’.
PERFORM write_text
USING ‘vom Tricktresor’ ‘TimesNewRoman’ ’10’ ”.
CALL METHOD OF h_word ‘InsertPara’.
CALL METHOD OF h_word ‘InsertPara’.
PERFORM write_text
USING ‘https://tricktresor.com’ ‘Courier’ ’10’ ”.
ENDFORM. ” ende
- IMG-Struktur anzeigen - 11. März 2024
- ALV-Grid und Dropdown - 8. März 2024
- Finden ─ nicht suchen - 28. Februar 2024